It has good coverage on Generics, Immutable Classes, Code Cohesion and protecting against change in your code base.
http://www.techartifact.com/blogs/2013/04/how-jre-works-internally.html/comment-page-1#comment-5028
The Java Web 2.0 Application Stack
Tips from the Bottom to the Top of the Stack.
Monday, April 29, 2013
Monday, April 15, 2013
Struts 2 sx:datetimepicker not setting the appropriate attribute in the action
One unobvious reason that the Struts2 DOJO datetimepicker may not be setting the appropriate attribute within your action may be because your theme of your s:form may be set to simple. Try not specifying the theme.
Thursday, March 29, 2012
Eclipse error : Failed to create the Java Virtual Machine
If you get this error one day, the fix is to simply add a pointer in eclipse.ini to a javaw.exe.
For example:
For example:
-vm
C:\JDK\jdk1.6.0_11\bin\javaw.exe
Tuesday, December 6, 2011
Win 7 Security 2012 Virus Infection
Recently a customer of mine had a virus on his computer called Win 7 Security 2012.
This Win 7 Security 2012 is a rogue anti-spyware application, which is often downloaded and installed by a Trojan, through browser security holes or via other unconventional unethical mechanisms. Once installed, Win 7 Security 2012 will display notifications of imaginary security and privacy risks in its attempts to get the user to purchase the full version and may generate system slowdown and instability. This program is extremely difficult to manually remove.
I noticed that this customer also had McAfee Security Center running and updated. I ran the scan and McAfee did not pick up this malware. Two thumbs down. I was able to find a free anti-virus to remove this virus available online.
If you are struggling with fixing this virus, let me know of your problems by commenting below and I'll be sure to get back to you.
Monday, December 5, 2011
Changing the Session Identifier (JSESSIONID) on Authentication. Protecting against Session Fixation attacks in Java Web Environments
It is a standard security practice to change the session identifier (JSESSIONID) after a successful login or authentication.
The attack scenario with this vulnerability is that a user can open a browser on a shared terminal and record the session identifier set by the application. Later when any other user of the system logs into the application without closing instances of that browser the same cookie will be used to track the victim's session.
Alternatively, if the application is susceptible to cross-site scripting on a publicly accessible page (most damagingly the home page), an attacker can use this vulnerability to learn the value of the session identifier, because the cookie does not change since it was first set. The attacker now knows the value of the session token can hijack the victim's session. This is a limited session fixation attack where the attacker does not have control over the value of the session identifier, but is able to know its value through various means before and after a user authenticates.
Most times, invalidating the session and creating a new one may suffice. However, if you are storing variables or objects, you may need to carry these variables or objects from the old session into the new session.
Below is a javax.servlet.Filter. This filter protects against the Session Fixation attacks described above. The filter looks for a specific session attribute, the (NEW_SESSION_INDICATOR) attribute. If one is found, the filter copies out relevant session data to a map, invalidates the session, creates a new session and loads the new session with the old session data.
The filter is simply mapped in your web.xml. Any place you successfully authenticate, an attribute is added to the session (NEW_SESSION_INDICATOR).
The code below follows:
Any questions about this posting or filter, comment below and I'll be sure to answer.
The attack scenario with this vulnerability is that a user can open a browser on a shared terminal and record the session identifier set by the application. Later when any other user of the system logs into the application without closing instances of that browser the same cookie will be used to track the victim's session.
Alternatively, if the application is susceptible to cross-site scripting on a publicly accessible page (most damagingly the home page), an attacker can use this vulnerability to learn the value of the session identifier, because the cookie does not change since it was first set. The attacker now knows the value of the session token can hijack the victim's session. This is a limited session fixation attack where the attacker does not have control over the value of the session identifier, but is able to know its value through various means before and after a user authenticates.
Most times, invalidating the session and creating a new one may suffice. However, if you are storing variables or objects, you may need to carry these variables or objects from the old session into the new session.
Below is a javax.servlet.Filter. This filter protects against the Session Fixation attacks described above. The filter looks for a specific session attribute, the (NEW_SESSION_INDICATOR) attribute. If one is found, the filter copies out relevant session data to a map, invalidates the session, creates a new session and loads the new session with the old session data.
The filter is simply mapped in your web.xml. Any place you successfully authenticate, an attribute is added to the session (NEW_SESSION_INDICATOR).
The code below follows:
import java.io.IOException; import java.util.Enumeration; import java.util.HashMap; import java.util.Map; import java.util.logging.Logger; import javax.servlet.Filter; import javax.servlet.FilterChain; import javax.servlet.FilterConfig; import javax.servlet.ServletException; import javax.servlet.ServletRequest; import javax.servlet.ServletResponse; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpSession; public class NewSessionFilter implements Filter { private static Logger logger = Logger.getLogger(NewSessionFilter.class.getName()); public static final String NEW_SESSION_INDICATOR = "filter.NewSessionFilter"; public void destroy() {} @SuppressWarnings("unchecked") public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException { if (request instanceof HttpServletRequest){ HttpServletRequest httpRequest = (HttpServletRequest) request; if (httpRequest.getSession(false) != null && httpRequest.getSession(false).getAttribute(NEW_SESSION_INDICATOR) != null ){ //copy session attributes from new session to a map. HttpSession session = httpRequest.getSession(); HashMapold = new HashMap (); Enumeration keys = (Enumeration ) session.getAttributeNames(); while (keys.hasMoreElements()) { String key = keys.nextElement(); if (!NEW_SESSION_INDICATOR.equals(key)) { old.put(key, session.getAttribute(key)); session.removeAttribute(key); } } logger.info("session invalidated on " + httpRequest.getRequestURI()); //invalidation session and create new session. session.invalidate(); session = httpRequest.getSession(true); //copy key value pairs from map to new session. for (Map.Entry entry : old.entrySet()) { session.setAttribute(entry.getKey(), entry.getValue()); } logger.info((new StringBuffer()).append("new Session for URI '") .append(httpRequest.getRequestURI()).append("':") .append( session.getId()).toString()); } } chain.doFilter(request, response); } public void init(FilterConfig filterconfig) {} }
Any questions about this posting or filter, comment below and I'll be sure to answer.
Wednesday, November 30, 2011
Steps to a CAPTCHA Implementation using JAVA/JSP
CAPTCHA can quickly and easily protect your web application against brute force and bot attacks or abuse. There are just a few simple steps to a CAPTCHA implementation in Java/JSP. The solution is simple and the documentation is quite clear, so I only provide the steps and quick links to those resources.
Step 1: Signup for a CAPTCHA account and generate keys for your website domains.
Navigate to http://www.google.com/recaptcha and signup for an account. After obtaining a login, generate keys for your domain.
Step 2: Find the developers guide for CAPTCHA
Navigate to http://code.google.com/apis/recaptcha/intro.html. From here, you'll find all the information you need. Notice in the left hand menu, there's a Java/JSP Plugin link available. Click into that.
Step 3: Download the Java/JSP Plugin and Implement
Navigate to http://code.google.com/apis/recaptcha/docs/java.html where you will find a link to download the plugin, which is a set of Java classes. Extract the source files into your web applications java source tree. The directions on the page are extremely straight forward.
The form page looks like:
By default the Java Virtual Machine (JVM) caches all DNS lookups forever instead of using the time-to-live (TTL) value which is specified in the DNS record of each host. To fix this issue for good, you can pass -Dsun.net.inetaddr.ttl=30 to your app-server (this tells Java to only cache DNS for 30 seconds).
There is a great article on the JVM and DNS caching. A must read at http://www.sdn.sap.com/irj/scn/weblogs?blog=/pub/wlg/1887
The steps above are quick and easy to implement, post back and let me know if you have any issues with the implementation and I will try and assist.
Step 1: Signup for a CAPTCHA account and generate keys for your website domains.
Navigate to http://www.google.com/recaptcha and signup for an account. After obtaining a login, generate keys for your domain.
Step 2: Find the developers guide for CAPTCHA
Navigate to http://code.google.com/apis/recaptcha/intro.html. From here, you'll find all the information you need. Notice in the left hand menu, there's a Java/JSP Plugin link available. Click into that.
Step 3: Download the Java/JSP Plugin and Implement
Navigate to http://code.google.com/apis/recaptcha/docs/java.html where you will find a link to download the plugin, which is a set of Java classes. Extract the source files into your web applications java source tree. The directions on the page are extremely straight forward.
The form page looks like:
<%@ page import="net.tanesha.recaptcha.ReCaptcha" %> <%@ page import="net.tanesha.recaptcha.ReCaptchaFactory" %> <html> <body> <form action="" method="post"> <% ReCaptcha c = ReCaptchaFactory.newReCaptcha("your_public_key", "your_private_key", false); out.print(c.createRecaptchaHtml(null, null)); %> <input type="submit" value="submit" /> </form> </body> </html>You also may be using reCaptcha over https. In that case, follow the instructions from this page: http://code.google.com/apis/recaptcha/docs/tips.html
<script type="text/javascript" src="https://www.google.com/recaptcha/api/challenge?k=your_public_key"> </script> <noscript> <iframe src="https://www.google.com/recaptcha/api/noscript?k=your_public_key" height="300" width="500" frameborder="0"></iframe><br> <textarea name="recaptcha_challenge_field" rows="3" cols="40"> </textarea> <input type="hidden" name="recaptcha_response_field" value="manual_challenge"> </noscript>When the form is submitted, the reCaptcha entries can be verified easily.
<%@ page import="net.tanesha.recaptcha.ReCaptchaImpl" %> <%@ page import="net.tanesha.recaptcha.ReCaptchaResponse" %> <html> <body> <% String remoteAddr = request.getRemoteAddr(); ReCaptchaImpl reCaptcha = new ReCaptchaImpl(); reCaptcha.setPrivateKey("your_private_key"); String challenge = request.getParameter("recaptcha_challenge_field"); String uresponse = request.getParameter("recaptcha_response_field"); ReCaptchaResponse reCaptchaResponse = reCaptcha.checkAnswer(remoteAddr, challenge, uresponse); if (reCaptchaResponse.isValid()) { out.print("Answer was entered correctly!"); } else { out.print("Answer is wrong"); } %> </body> </html>Step 4: Give the JVM a time interval to refresh its DNS cache
By default the Java Virtual Machine (JVM) caches all DNS lookups forever instead of using the time-to-live (TTL) value which is specified in the DNS record of each host. To fix this issue for good, you can pass -Dsun.net.inetaddr.ttl=30 to your app-server (this tells Java to only cache DNS for 30 seconds).
There is a great article on the JVM and DNS caching. A must read at http://www.sdn.sap.com/irj/scn/weblogs?blog=/pub/wlg/1887
The steps above are quick and easy to implement, post back and let me know if you have any issues with the implementation and I will try and assist.
Thursday, November 17, 2011
Disabling certain HTTP Methods in Tomcat
HTTP protocol defines eight methods that can be performed on a resource on the HTTP server. GET, POST and HEAD are the most common methods that are used to access information provided by a web server. The other methods such as OPTIONS, PUT, DELETE, CONNECT and TRACE are not normally used in the general operation of a web server can potentially pose a security risk for any web application. So it is good practice to restrict the response to specific HTTP Methods.
First, determine which HTTP Methods your installation is responding too. I use browser plug-ins that enable me to submit HTTP requests, specifying the URL and HTTP method. There are various plugins available for Chrome and Firefox and I do not make any recommendations here.
Second, according to your test results, configure your Tomcat installtion to not respond for certain HTTP Methods. This can be configured at the instance level by inserting a <security-constraint> element directly under the <web-app> element, in the installations web.xml file located at.
[tomcatinstallation]/conf/web.xml
Below is the added configuration.
The configuration above will disable the HTTP Methods TRACE, PUT, OPTIONS or DELETE.
Any questions, comment and I'll be sure to answer them.
First, determine which HTTP Methods your installation is responding too. I use browser plug-ins that enable me to submit HTTP requests, specifying the URL and HTTP method. There are various plugins available for Chrome and Firefox and I do not make any recommendations here.
Second, according to your test results, configure your Tomcat installtion to not respond for certain HTTP Methods. This can be configured at the instance level by inserting a <security-constraint> element directly under the <web-app> element, in the installations web.xml file located at.
[tomcatinstallation]/conf/web.xml
Below is the added configuration.
<security-constraint>
<web-resource-collection>
<web-resource-name>restricted methods</web-resource-name>
<url-pattern>/*</url-pattern>
<http-method>TRACE</http-method>
<http-method>PUT</http-method>
<http-method>OPTIONS</http-method>
<http-method>DELETE</http-method>
</web-resource-collection>
<auth-constraint />
</security-constraint>
The configuration above will disable the HTTP Methods TRACE, PUT, OPTIONS or DELETE.
Any questions, comment and I'll be sure to answer them.
Thursday, November 3, 2011
javax.net.ssl.SSLException: Received fatal alert: unexpected_message
If you are attempting to establish an SSL Connection as a client to a server and getting this error at the very end of the SSL Handshake, then check the server settings for client authentication.
Client Authentication is the ability of a webserver to verify the client, whether it be a browser or other application.
Setting client authentication does reduce the level of security enabled, so this decision should be made based on your needs and threat model.
If you do require client authentication, there are two great articles below:
If you have any JSSE/OpenSSL questions, feel free to comment and I'll try and get back to you.
Client Authentication is the ability of a webserver to verify the client, whether it be a browser or other application.
Setting client authentication does reduce the level of security enabled, so this decision should be made based on your needs and threat model.
If you do require client authentication, there are two great articles below:
If you have any JSSE/OpenSSL questions, feel free to comment and I'll try and get back to you.
SSL Connections over a proxy using JSSE
The Java Secure Socket Extension (JSSE) enables secure Internet communications. It provides a framework and an implementation for a Java version of the SSL and TLS protocols and includes functionality for data encryption, server authentication, message integrity, and optional client authentication. Using JSSE, developers can provide for the secure passage of data between a client and a server running any application protocol, such as Hypertext Transfer Protocol (HTTP), Telnet, or FTP, over TCP/IP.
The https protocol is similar to http, but https first establishes a secure channel via SSL/TLS sockets and then verifies the identity of the peer before requesting/receiving data. javax.net.ssl.HttpsURLConnection extends the java.net.HttpsURLConnection class, and adds support for https-specific features. Upon obtaining a HttpsURLConnection, you can configure a number of http/https parameters before actually initiating the network connection via the method URLConnection.connect.
In some situations, it is desirable to specify the SSLSocketFactory that an HttpsURLConnection instance uses. For example, you may wish to tunnel through a proxy type which is NOT supported by the default implementation. The new SSLSocketFactory could return sockets that have already performed all necessary tunneling, thus allowing HttpsURLConnection to use additional proxies.
Post your issue below, and I'll try and answer your JSSE questions.
References:
The https protocol is similar to http, but https first establishes a secure channel via SSL/TLS sockets and then verifies the identity of the peer before requesting/receiving data. javax.net.ssl.HttpsURLConnection extends the java.net.HttpsURLConnection class, and adds support for https-specific features. Upon obtaining a HttpsURLConnection, you can configure a number of http/https parameters before actually initiating the network connection via the method URLConnection.connect.
In some situations, it is desirable to specify the SSLSocketFactory that an HttpsURLConnection instance uses. For example, you may wish to tunnel through a proxy type which is NOT supported by the default implementation. The new SSLSocketFactory could return sockets that have already performed all necessary tunneling, thus allowing HttpsURLConnection to use additional proxies.
Post your issue below, and I'll try and answer your JSSE questions.
References:
Saturday, August 27, 2011
Photo Card
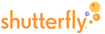
Jungle Favor Birthday
Shop birthday cards and invitations at Shutterfly.com.
View the entire collection of cards.
Subscribe to:
Posts (Atom)